Mock GraphQL API using json-graphql-server
In the previous blog posts, I have covered how we can develop GraphQL API using Netflix DGS framework.
If the GraphQl API is used by any front end application or any other GraphQL client, the developer has to wait for the complete API to implement. One way to overcome the problem is to mock the GraphQL API, So that the developers can continue his work till the actual API is completely ready.
In this blog post I will cover, how we can mock the GraphQL API using json-graphql-server
without implementing actual API.
Required software
- Node JS
- NPM
1) Using json-grpahql-server
Using the json-graphql-server
npm package, we can set up the mock GraphQL server in few minutes with zero configuration.
For setting up GraphQL server, all you need is just prepare the json data as per your schema and install the npm package.
Installation
npm install -g json-graphql-server
Code language: JavaScript (javascript)
Data preparation
create db.json file with data.
data file should export an object where the keys are the entity types. The values should be lists of entities, i.e. arrays of value objects with at least an id
key
module.exports = { departments: [ { id: 1, dept_name: "Lorem Ipsum" }, { id: 2, dept_name: "Sic Dolor amet" }, ], employees: [ { id: 123, first_name: "John Doe", last_name: "Last", gender: "M", birth_date: new Date("2017-07-03"), hire_date: new Date("2017-09-03"), department_id: 1, }, { id: 456, first_name: "John 456", last_name: "Last", gender: "M", birth_date: new Date("2017-07-03"), hire_date: new Date("2017-07-03"), department_id: 1, }, { id: 789, first_name: "John 789", last_name: "Last", gender: "M", birth_date: new Date("2017-07-03"), hire_date: new Date("2017-07-03"), department_id: 2, }, ], };
Note
While preparing the data , we need to follow certain conventions so that json-graphql-server
can establish relationships properly
1. Always define entity names as plural (Ex: departments, employees)
2. For establishing relationship use singular entity name . (Ex: To establish relationship between employees and departments , we are referring id of department in employee object as department_id.
3.Also, make sure that you use the same case for entities and referring ids ( Ex : departments -> department_id , do not write as Department_id)
Start the GraphQL Server
Now you can start the GraphQL server with following command
json-graphql-server db.js
By default, GraphQl server starts localhost on port 3000

To use a port other than 3000, you can run json-graphql-server db.js --p <choosen port here>

To use a host other than localhost, you can run json-graphql-server db.js --h <choosen host here>
Note : Before changing the host name , you need to update your host file so that your new host name points to 127.0.0.1 address

The server is GraphiQL enabled, so you can query your server using a full-featured graphical user interface, providing autosuggest, history, etc.
You can point your browser to http://localhost:3000/grpahiql to open the GUI client.
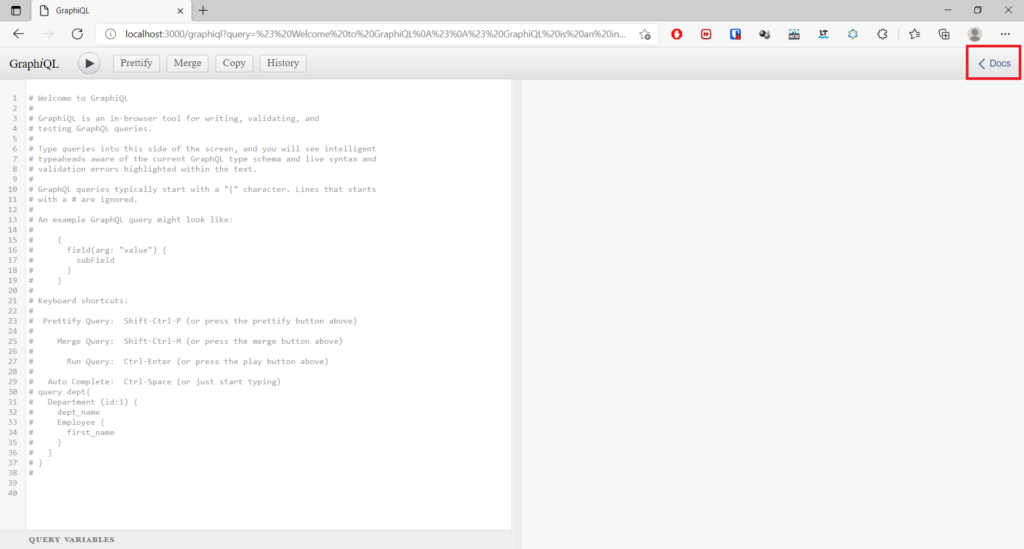
By clicking on the Docs
, we can view generated Queries and Mutations
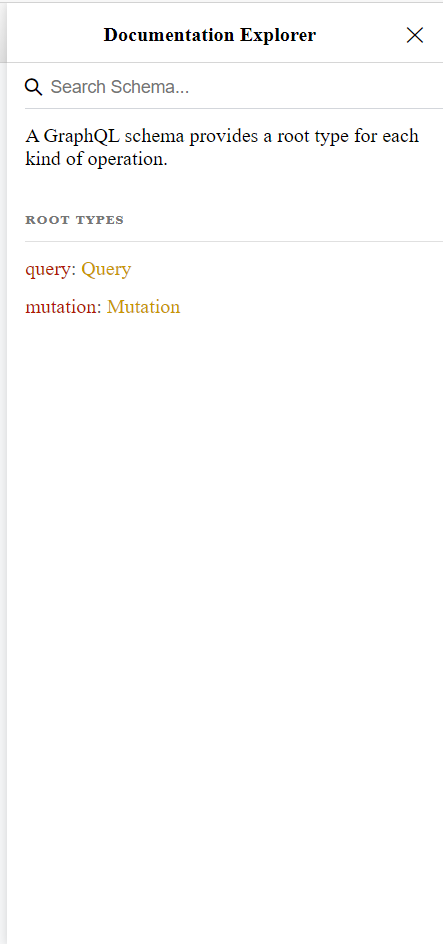
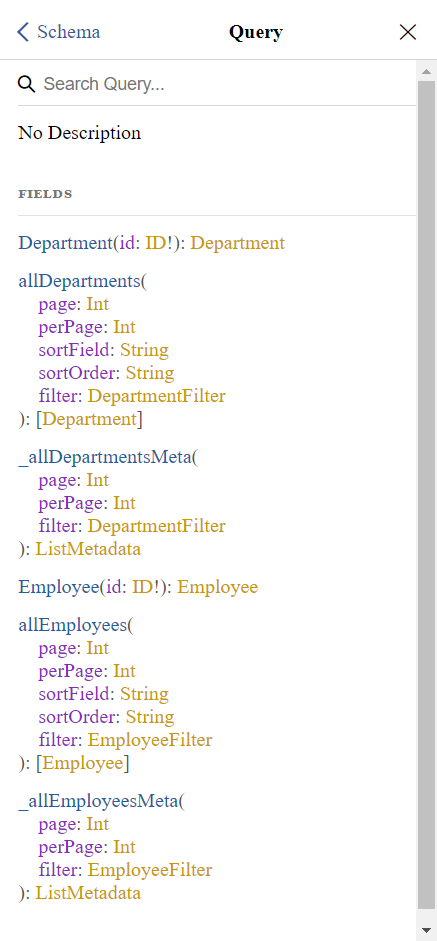
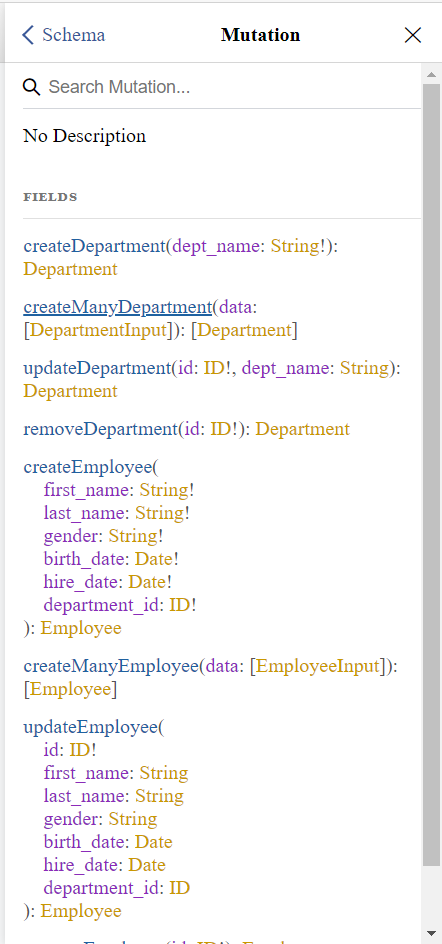
Based on your data, json-graphql-server will generate a schema with one type per entity, as well as 3 query types and 3 mutation types. You can view them in the above screenshots.
Test the GraphQL API
Now Let’s test some Query and Mutation types
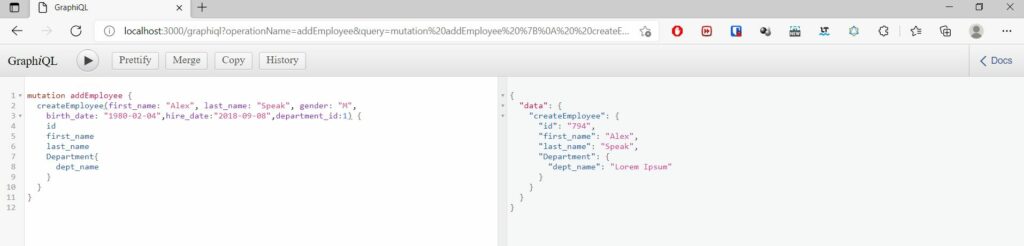

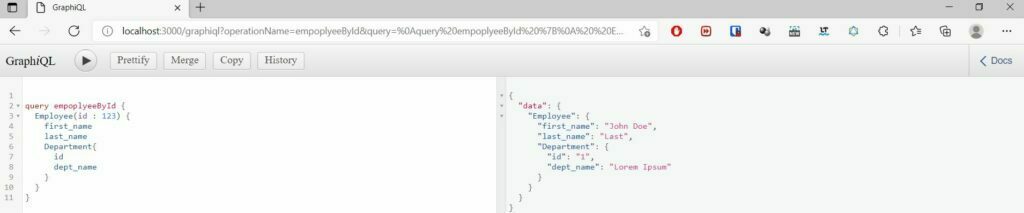
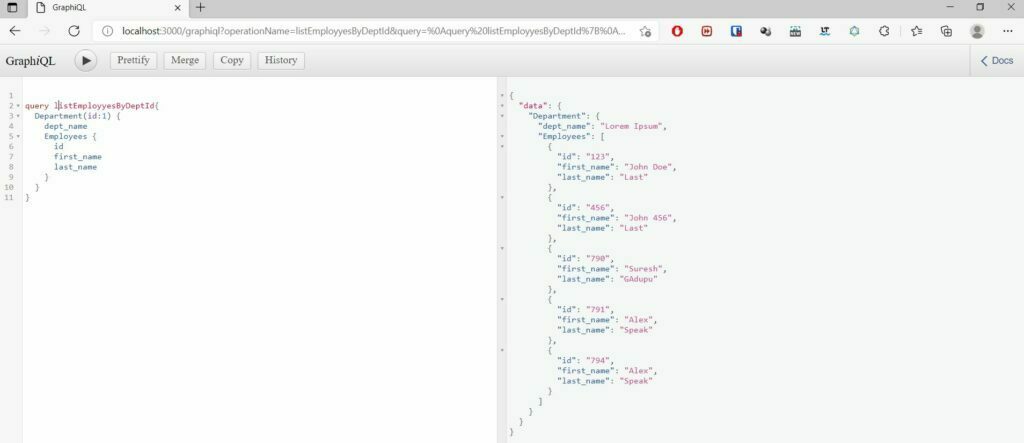
Mock with Node JS
We can also start mock server with node js program
Create a directory
mkdir node-graphql-mock-server
Code language: JavaScript (javascript)
Create package.json file
npm init -y
Code language: JavaScript (javascript)
To enable module support, add the following text in package.json file
"type": "module"
Code language: Java (java)
Install json-graphql-server package
npm install --save-dev json-graphql-server
Code language: JavaScript (javascript)
Create index.js file and following code
import express from 'express';
import jsonGraphqlExpress from 'json-graphql-server';
import cors from 'cors';
const HOST = "localhost";
const PORT = 3000;
const app = express();
const data = {
departments: [
{ id: 1, dept_name: "Lorem Ipsum" },
{ id: 2, dept_name: "Sic Dolor amet" },
],
employees: [
{
id: 123,
first_name: "John Doe",
last_name: "Last",
gender: "M",
birth_date: new Date("2017-07-03"),
hire_date: new Date("2017-09-03"),
department_id: 1,
},
{
id: 456,
first_name: "John 456",
last_name: "Last",
gender: "M",
birth_date: new Date("2017-07-03"),
hire_date: new Date("2017-07-03"),
department_id: 1,
},
{
id: 789,
first_name: "John 789",
last_name: "Last",
gender: "M",
birth_date: new Date("2017-07-03"),
hire_date: new Date("2017-07-03"),
department_id: 2,
},
],
};
app.use(cors());
app.use('/graphql', jsonGraphqlExpress.default(data));
app.listen(PORT,HOST);
let msg = `GraphQL server running with your data at http://${HOST}:${PORT}/`;
console.log(msg);
Code language: JavaScript (javascript)
Now you can start the mock server with following command from command promt
node index.js
Code language: JavaScript (javascript)

You can download complete source code from GitHub
You can point your browser to http://localhost:3000/grpahql to open the GUI client.
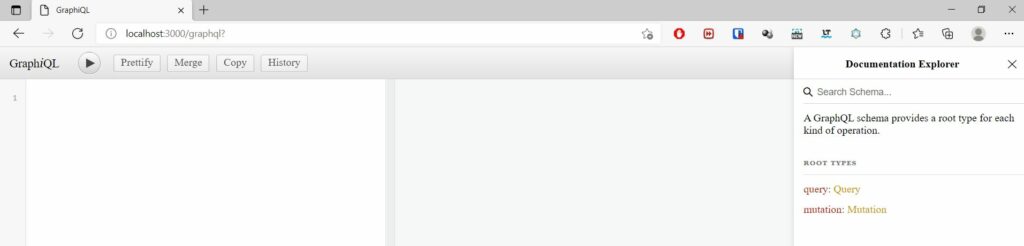
Major advantages of using json-graphql-server are zero configurations and just need to prepare the data to start the mocking.
Coming to disadvantages, you can not define your own custom query or mutation types.