Creating React JS App using Vite 2.0

In this blog post I will explain what is Vite and how you can create new react js app using Vite
It consists of two major parts
- A dev server that provides extremely fast Hot Module Replacement (HMR)
- A build command that bundles your code with Rollup
Prior to the availability of ES modules in browsers, developers did not have a native mechanism for building JavaScript in a modular way. Therefore, we are familiar with the concept of “bundles”. That is, use tools that crawl, process, and bind source modules into files that you can run in your browser.
Over time we have seen tools like webpack, Rollup and Parcel, which greatly improved the development experience for frontend developers.
However, when we begin to construct more ambitious apps, the quantity of JavaScript we must deal with grows dramatically. Thousands of modules are not unusual in large-scale projects. We are approaching a speed bottleneck for JavaScript-based tooling: it may frequently take an unreasonable amount of time (up to minutes!) to fire up a dev server, and even with HMR, file updates can take a few seconds to be reflected in the browser. The sluggish feedback loop can have a significant impact on developer productivity and pleasure.
Vite aims to address these issues by leveraging new advancements in the ecosystem: the availability of native ES modules in the browser, and the rise of JavaScript tools written in compile-to-native languages.
Vite doesn’t bundle the code in advance. Instead, when the browser asks for a file, Vite transforms it.
Vite Features
- On demand file serving over native ESM
- Hot Module Replacement (HMR)
- Out-of-the-box support for TypeScript, JSX, CSS and more
- Optimized build with Rollup JS
- Plugin support
- Fully Typed APIs with full TypeScript
[icon name=”clipboard” prefix=”fas”] Note
Vite requires Node.js version >=12.0.0
Installing Vite
you can install Vite using below commands
With NPM:
npm init vite@latest
Code language: Makefile (makefile)

With Yarn:
yarn create vite
Code language: Shell Session (shell)
With PNPM
pnpm create vite
Code language: Shell Session (shell)
Following the prompt you can create a project.
You can also directly specify the project name and the template you want to use via additional command line options
Vite currently supports below framework templates.
vanilla
vanilla-ts
vue
vue-ts
react
react-ts
preact
preact-ts
lit
lit-ts
svelte
svelte-ts
Creating React JS App using Vite
For creating React JS app with Vite
you can use below command line options.
# npm 6.x
npm create vite@latest my-react-app --template react
# If you want to create react project with typescript
npm create vite@latest my-react-app --template react-ts
# npm 7+, extra double-dash is needed:
npm create vite@latest my-react-app -- --template react
# yarn
yarn create vite my-react-app --template react
# pnpm
pnpm create vite my-react-app -- --template react
Code language: Java (java)
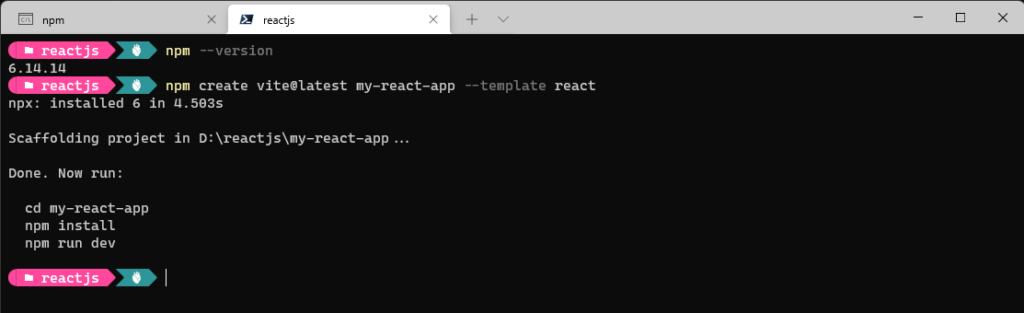
Now change to project directory and run below command to install the npm packages
npm install
Code language: Shell Session (shell)
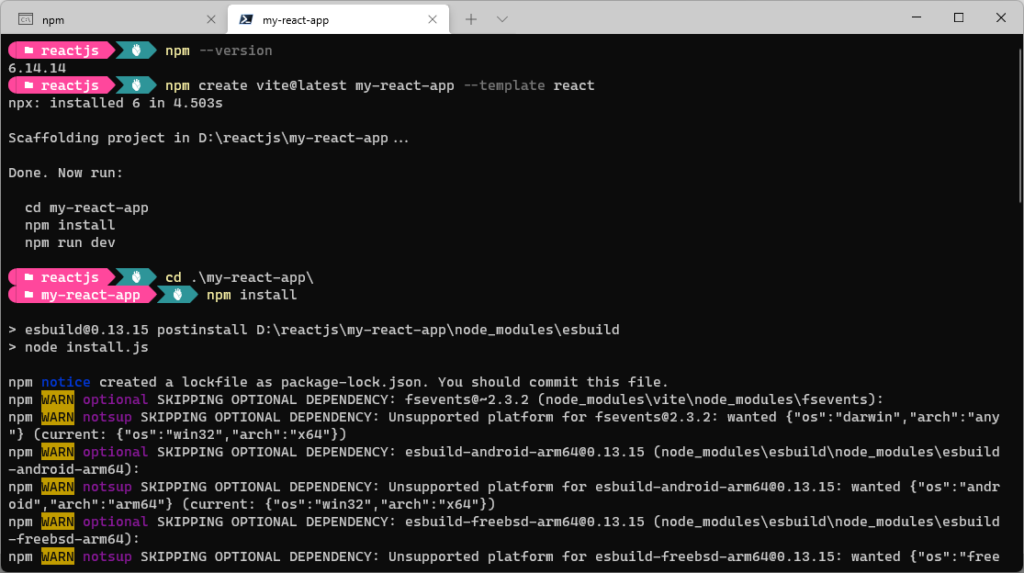
After installing dependencies, you can start the application with following command
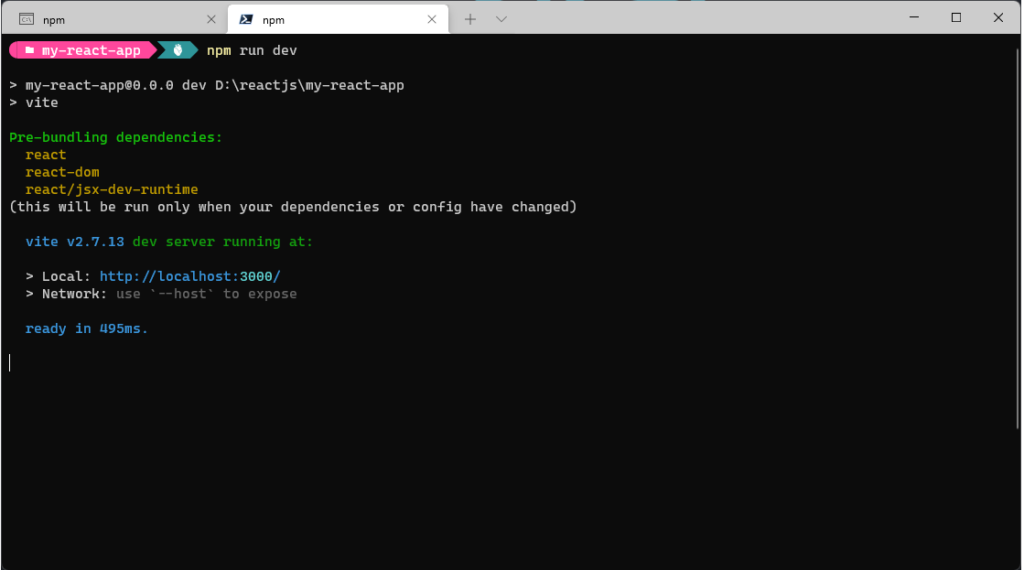
Now open your browser, and point it to http://localhost:3000
address
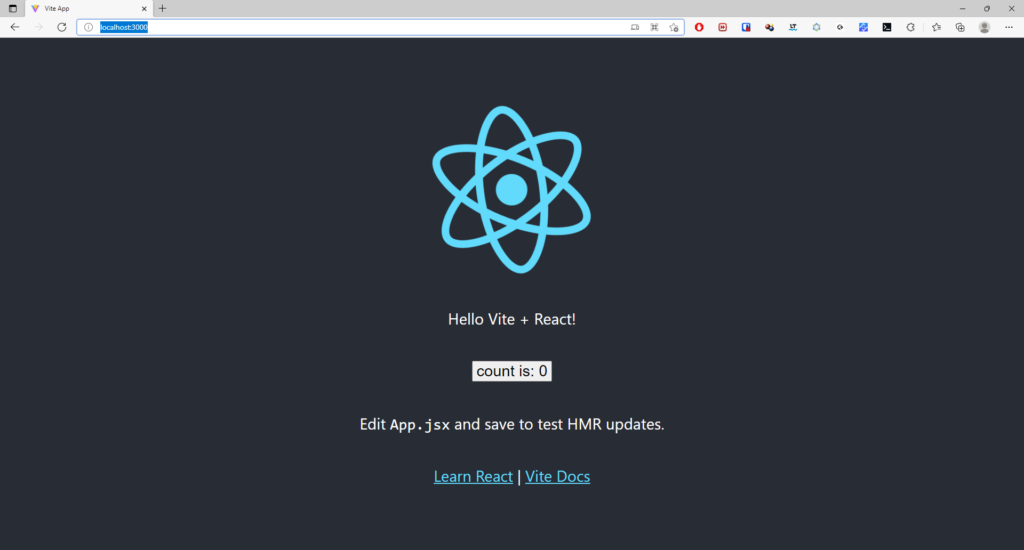
The whole project scaffolding process takes less than1 minute.
Trying Vite Online
You can try Vite online on StackBlitz. It runs the Vite-based build setup directly in the browser
Vite Resources
You can find community curated plugins and templates for various frameworks in following Git repo