Enforce Coding Standards with Maven Checkstyle Plugin
Maintaining a clean, consistent, and readable code base is essential for every software development project. One powerful tool to help achieve this goal is the Maven Checkstyle Plugin. This article explores the basics of the plugin, its configurations, and benefits.
What is Maven Checkstyle Plugin?
The Maven Checkstyle Plugin is an essential code quality tool that enables developers to enforce coding standards and conventions across a project automatically. By integrating this plugin into your build process, you ensure that your code adheres to specific formatting guidelines, improving readability and maintainability.
Why Use Maven Checkstyle Plugin?
1. Enforces coding standards: The plugin verifies that the code follows predefined rules, making it easier to maintain a uniform coding style throughout the project.
2. Increases code readability: With consistent styling in place, developers can more easily understand each other’s code, reducing confusion and speeding up collaboration.
3. Simplifies code reviews: Automatic checking of style rules means less time spent on nitpicking minor style issues during code reviews.
The plugin can be configured to check for various coding conventions, such as:
- Indentation and spacing
- Naming conventions
- Comments and documentation
- Code organization and structure
Predefined Formatting Rule Sets
There are 2 predefined Checkstyle configuration definitions that ship with the plugin, the Sun Microsystems Definition is used by default.
- sun definitions(sun_checks.xml)
- google definitions(google_checks.xml)
You can also define your own rule sets and apply them.
How to Set Up Maven Checkstyle Plugin:
In pom.xml add the following configuration in plugins section.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.3.0</version>
<configuration>
<configLocation>google_checks.xml</configLocation>
<consoleOutput>true</consoleOutput>
<failsOnError>true</failsOnError>
</configuration>
<executions>
<execution>
<id>validate</id>
<phase>validate</phase>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
Code language: Java (java)
In above configuration checks whether java files formatted according google java styles and we also configured to fail the build if there are check style errors.
I tried to build project from repo https://github.com/sureshgadupu/springboot-resource-file using above configuration file.
mvn clean build
Code language: Java (java)
....
[INFO] --- maven-checkstyle-plugin:3.3.0:check (validate) @ springboot-resource-file ---
[INFO] Starting audit...
.....
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:70:5: Missing a Javadoc comment. [MissingJavadocMethod]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:72: Line is longer than 100 characters (found 119). [LineLength]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:72:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:74:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:75:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:76:5: 'method def rcurly' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:78:5: 'method def modifier' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:80:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:81:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:82:5: 'method def rcurly' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:84:5: 'method def modifier' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:86:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:87:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:88:5: 'method def rcurly' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:90:5: 'method def modifier' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:91:19: Abbreviation in name 'readPDF' must contain no more than '1' consecutive capital letters. [AbbreviationAsWordInName]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:92:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:93:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:94:5: 'method def rcurly' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:96:5: 'method def modifier' has incorrect indentation level 4, expected level should be 2. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:96:5: Missing a Javadoc comment. [MissingJavadocMethod]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:98:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:100:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:101:9: 'method def' child has incorrect indentation level 8, expected level should be 4. [Indentation]
[WARN] D:\IdeaProjects\springboot-resource-file\src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:102:5: 'method def rcurly' has incorrect indentation level 4, expected level should be 2. [Indentation]
Audit done.
[INFO] You have 0 Checkstyle violations.
....
[INFO] --- spring-boot-maven-plugin:3.1.2:repackage (repackage) @ springboot-resource-file ---
[INFO] Replacing main artifact D:\IdeaProjects\springboot-resource-file\target\springboot-resource-file-0.0.1-SNAPSHOT.jar with repackaged archive, adding nested dependencies in BOOT-INF/.
[INFO] The original artifact has been renamed to D:\IdeaProjects\springboot-resource-file\target\springboot-resource-file-0.0.1-SNAPSHOT.jar.original
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 24.971 s
[INFO] Finished at: 2023-08-29T23:47:17+12:00
Code language: Java (java)
In above console log you can see that, build was successful even though there were warnings about check style issues.
By default severity level set to error. so check style plugin did not fail the build.
To fail the build when there are check style warning we need to use <violationSeverity> element in configuration and set it to warning.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.3.0</version>
<configuration>
<configLocation>google_checks.xml</configLocation>
<consoleOutput>true</consoleOutput>
<failsOnError>true</failsOnError>
<violationSeverity>warning</violationSeverity>
</configuration>
<executions>
<execution>
<id>validate</id>
<phase>validate</phase>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
Code language: Java (java)
If you build the project again it will fail the build.
mvn clean package
Code language: Java (java)
....
[WARNING] src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:[98,9] (indentation) Indentation: 'method def' child has incorrect indentation level 8, expected level should be 4.
[WARNING] src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:[100,9] (indentation) Indentation: 'method def' child has incorrect indentation level 8, expected level should be 4.
[WARNING] src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:[101,9] (indentation) Indentation: 'method def' child has incorrect indentation level 8, expected level should be 4.
[WARNING] src\main\java\dev\fullstackcode\resource\file\example\controller\WelcomeController.java:[102,5] (indentation) Indentation: 'method def rcurly' has incorrect indentation level 4, expected level should be 2.
[INFO] ------------------------------------------------------------------------
[INFO] BUILD FAILURE
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 4.015 s
[INFO] Finished at: 2023-08-30T00:15:26+12:00
Code language: Java (java)
Checckstyle plugin showing warnings about indentation , line length and missing Javadoc comments.
To fix the check style issues, either you can do manually or use plugins developed for your favorite IDEs.
Google java format plugin is available for both IntelliJ Idea and VS Code, which automatically formats when ever you save the file.
If you are using IntelliJ Idea, you can go to File -> Settings -> Plugin
In Market place search for google-java-format plugin , install it and enable the plugin for your project.
You can enable the google java format plugin by going File -> Settings -> Other Settings
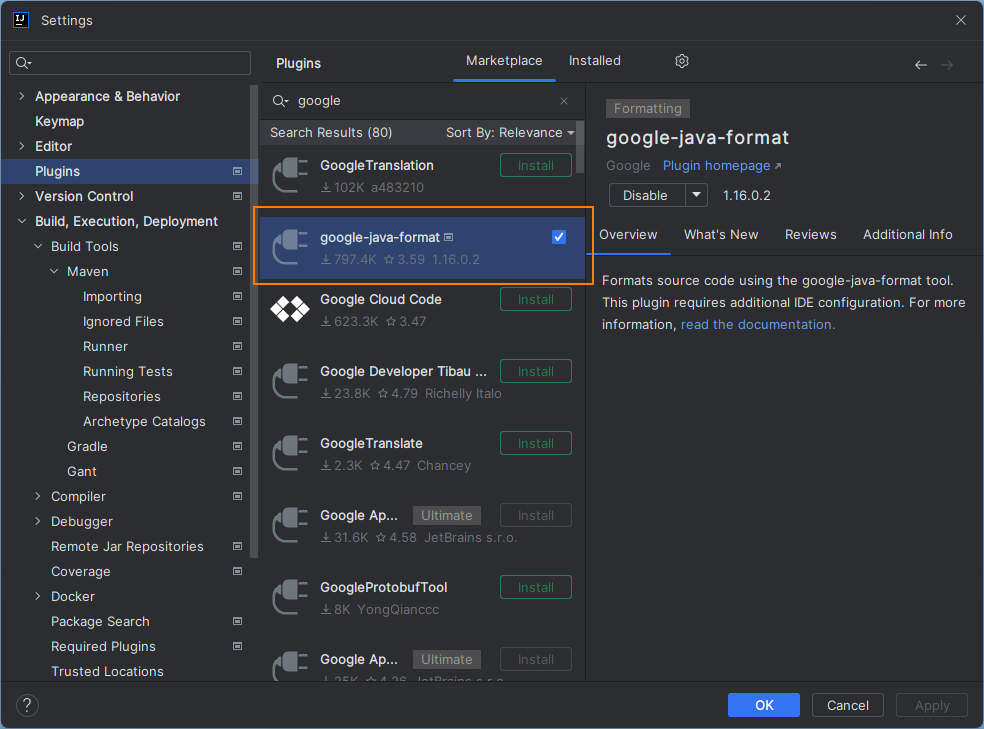
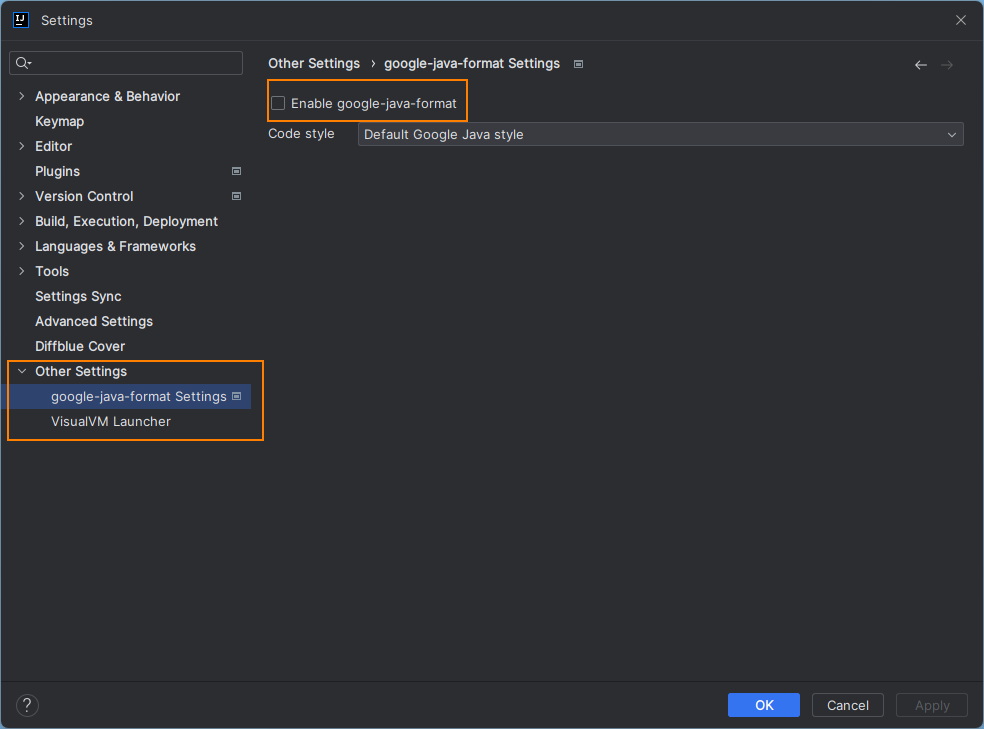
Once re-formatted all the java files and fixed the all the check-style issues build was successful.
[INFO] ----< dev.fullstackcode.resource.example:springboot-resource-file >-----
[INFO] Building springboot-resource-file 0.0.1-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- maven-checkstyle-plugin:3.3.0:check (default-cli) @ springboot-resource-file ---
[INFO] Starting audit...
Audit done.
[INFO] You have 0 Checkstyle violations.
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 2.621 s
[INFO] Finished at: 2023-08-30T23:27:34+12:00
[INFO] ------------------------------------------------------------------------
Code language: Java (java)
If you to just check the style violations with out building the entire project, you can use following command.
mvn checkstyle:check
Code language: Java (java)
Applying Checkstyle rules to Test Classes
By default Checkstyle plugin applies rules to only source classes. If you want to apply formatting rules to test classes add <includeTestSourceDirectory> tag inside configuration.
<configuration>
...
<includeTestSourceDirectory>true</includeTestSourceDirectory>
</configuration>
Code language: Java (java)
Applying Checkstyle rules to Resource Files
you can also apply the formatting rules to resource files by using <includeResources> and <includeTestResources> tags
<configuration>
...
<includeResources>true</includeResources>
<includeTestResources>true</includeTestResources>
...
</configuration>
Code language: Java (java)
Specifying source directories
By default, Checkstyle plugin assumes that sources are in src/main/java and src/main/test. If you want specify custom directory structure then you have use <sourceDirectories> tag to specify source directories.
When you use <sourceDirectories> tag, you have to explicitly specify all the source directories
<configuration>
....
<sourceDirectories>
<sourceDirectory>src/main/java</sourceDirectory>
<sourceDirectory>src/test/java</sourceDirectory>
<sourceDirectory>src/integrationtest/java</sourceDirectory>
</sourceDirectories>
.....
</configuration>
Code language: Java (java)
Specify Filter For Source Files
By default, Checkstyle plugin checks the formatting rules for files with .java extension.If you want include files with other extensions then you to specify them in <includes> tag.
<configuration>
...
<includes>**\/*.java, **\/*.properties</includes>
...
<configuration>
Code language: Java (java)
Generate Checkstyle Report As Part of the Project Reports
The initial checkstyle plugin setup we configured in blog post is used at the build time. If you want to generate report as part of the project reports you have to use below configuration.
<project>
...
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.3.0</version>
<reportSets>
<reportSet>
<reports>
<report>checkstyle</report>
</reports>
</reportSet>
</reportSets>
</plugin>
</plugins>
</reporting>
...
</project>
<a href="https://maven.apache.org/plugins/maven-checkstyle-plugin/usage.html#generate-checkstyle-report-as-part-of-the-project-reports"></a>
Code language: Java (java)
Then run following command to generate report
mvn site
Code language: Java (java)
Upgrading Checkstyle at Runtime
Maven Checkstyle plugin comes with a default Checkstyle version: for maven-checkstyle-plugin 3.3.0
, Checkstyle 9.3 is used by default.
Checkstyle versions often maintain high compatibility, you can modify the version used at runtime to take advantage of Checkstyle’s most recent bugfixes you need to add following configuration
<project>
...
<build>
<pluginManagement>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.3.0</version>
<dependencies>
<dependency>
<groupId>com.puppycrawl.tools</groupId>
<artifactId>checkstyle</artifactId>
<version>...choose your version...</version>
</dependency>
</dependencies>
</plugin>
</plugins>
</pluginManagement>
</build>
...
</project>
Code language: Java (java)